Node.js is a framework built upon the JavaScript Engine “V8“, which is currently the most used JavaScript Engine and built into Google Chrome.
Node.js was developed by the need of a resource efficient, performant, “nonblocking I/O” Framework to deliver fast and quick websites.
Here is an example of a very basic web-server written in Node.js
const http = require('http');
const hostname = '127.0.0.1';
const port = 3000;
const server = http.createServer((req, res) => {
res.statusCode = 200;
res.setHeader('Content-Type', 'text/plain');
res.end('Hello World\n');
});
server.listen(port, hostname, () => {
console.log(`Server running at http://${hostname}:${port}/`);
});
Main requirement to run this code is the fact, that you need a Node.js version installed on your system (see https://nodejs.org)
The current LTS version is fine.
Now we can start the webserver with
node index.js
and can see the following output in the terminal
Server running at http://127.0.0.1:3000/
If we open up a browser with the given URL we see
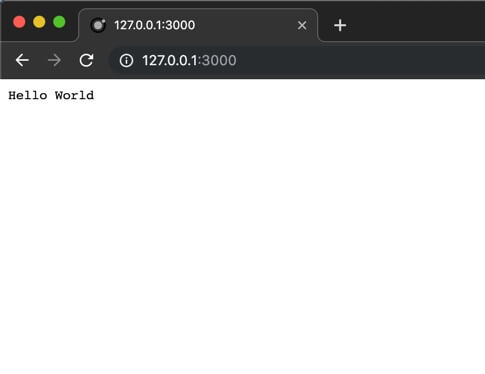
Advantages of Node.js
- Based on JavaScript there is very little to no new syntax to lean if you know the basics of JavaScript.
- Node.js is event based (see HERE for more detials) and because of that very performant
- Modularity with the Node-Package-Manager (NPM)
Disadvantages of Node.js
- Backwards compatibility is somewhat a problem with upcoming Node.js version updates because the current development of the framework is so rapid.
- The fact that Node.js works on an event loop implies only 1 thread has to carry all the work which can be overwhelmed by lots of requests and/or server logic.
- Asynchron code notation can be tricky to understand and learn
Source: https://nodejs.org/de/about/