Table of Contents
JavaScript (JS) is a Scripting language which is used for interactive browser elements as well as server logic.
Versions of JavaScript
The official name of JavaScript is “ECMAScript”.
Since 2015 the versioning system has changed from adding just 1, 2, 3 to ECMAScript and instead add the year of release.
This means ES6 = ECMAScript 2015 | ES7 = ECMAScript 2016 etc.
Ver | Official Name | Description |
---|---|---|
1 | ECMAScript 1 (1997) | First Edition. |
2 | ECMAScript 2 (1998) | Editorial changes only. |
3 | ECMAScript 3 (1999) | Added Regular Expressions. Added try/catch. |
4 | ECMAScript 4 | Never released. |
5 | ECMAScript 5 (2009) | Added “strict mode”. Added JSON support. Added String.trim(). Added Array.isArray(). Added Array Iteration Methods. |
5.1 | ECMAScript 5.1 (2011) | Editorial changes. |
6 | ECMAScript 2015 | Added let and const. Added default parameter values. Added Array.find(). Added Array.findIndex(). |
7 | ECMAScript 2016 | Added exponential operator (**). Added Array.prototype.includes. |
8 | ECMAScript 2017 | Added string padding. Added new Object properties. Added Async functions. Added Shared Memory. |
9 | ECMAScript 2018 | Added rest / spread properties. Added Asynchronous iteration. Added Promise.finally(). Additions to RegExp. |
10 | ECMAScript 2019 | Added Array.flat(). Added Object.fromEntries(). Added String.trimStart() & trimEnd(). Added Symbol.description. |
11 | ECMAScript 2020 | Added BigInt Typ. Added globalThis. Added Nullish Coalescing Operator (??). Added Optional Chaining Operator (?.). |
These versions are “just” the description of how the programming language should behave. The implementation of these behaviours are delivered by JavaScript Engines like:
- V8 is the most common JS Engine used by:
- Chrome/Chromium
- Node.js and Deno
- Edge
- SpiderMonkey used by Firefox
- Nitro used by Safari
- Chakra used by Internet-Explorer
Browser Support (ES6)
Browser | Version | Date |
---|---|---|
Chrome | 51 | Mai 2016 |
Firefox | 54 | Juni 2017 |
Edge | 14 | Aug 2016 |
Safari | 10 | Sep 2016 |
Opera | 38 | Juni 2016 |
Internet-Explorer is the only browser wich does not support ES6.
Clientside JS (Browser)
Clientside JS is being executed on each device which e.g. has your website open.
In Browsers JS is used to for example adjust the DOM without reloading the page.
document.addEventListener("DOMContentLoaded", function() {
function createParagraph() {
let para = document.createElement('p');
para.textContent = 'You clicked the button!';
document.body.appendChild(para);
}
const buttons = document.querySelectorAll('button');
for(let i = 0; i < buttons.length ; i++) {
buttons[i].addEventListener('click', createParagraph);
}
});
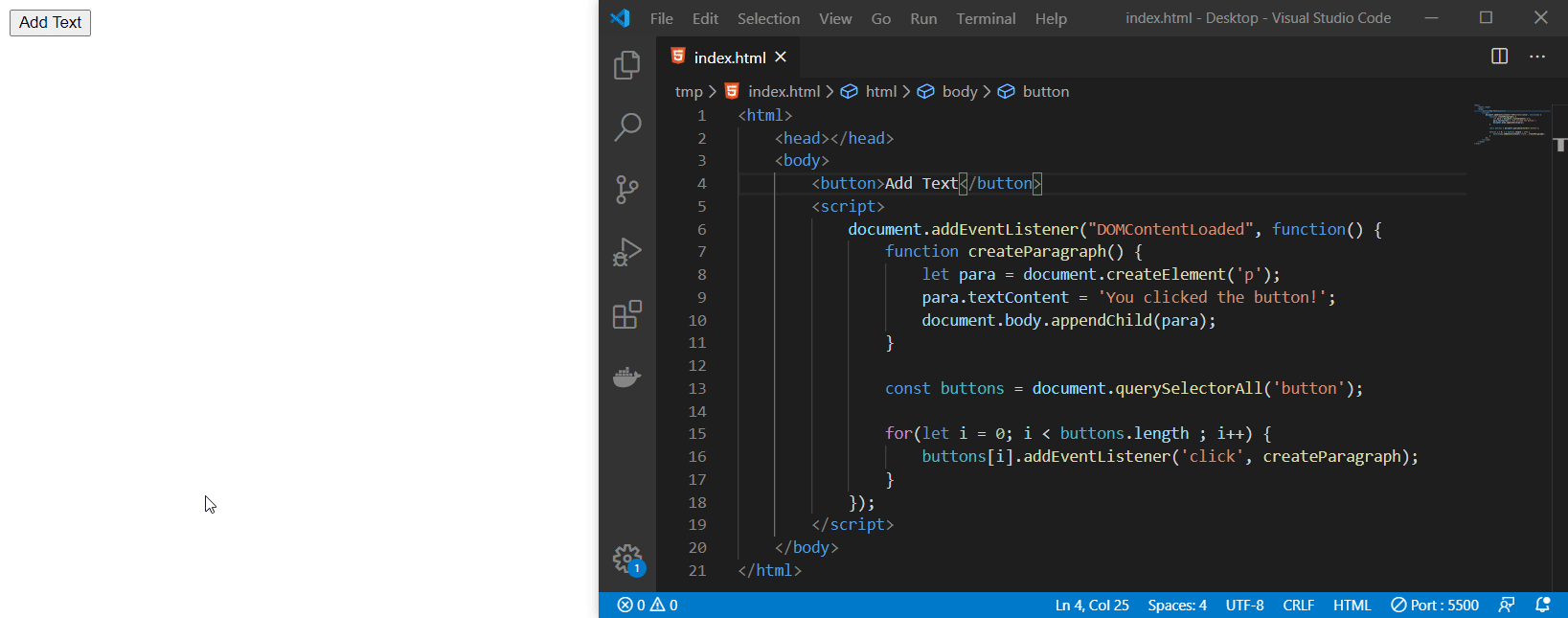
Via the JS Object “document” you can check, adjust and add elements shown in your website.
But there also some other APIs available as well, such as:
- Geo Location (navigator.geolocation) to get the current location of the user
- WebGL and Canvas API to create complex animations or 3D elements
- Audio & Video API
Serverside JS (Node.js)
Serverside JS is executed on the server to e.g. send text back to a client.
There are many frameworks which make it easier to create modern JS based websites and/or backends.
For more information on Node.js see HERE.
Source:
https://www.w3schools.com/js/js_versions.asp
https://developer.mozilla.org/en-US/docs/Learn/JavaScript/First_steps/What_is_JavaScript